A Guide to Using Sidekiq for Background Jobs in Ruby on Rails
Posted at 4-October-2024 / Written by Rohit Bhatt
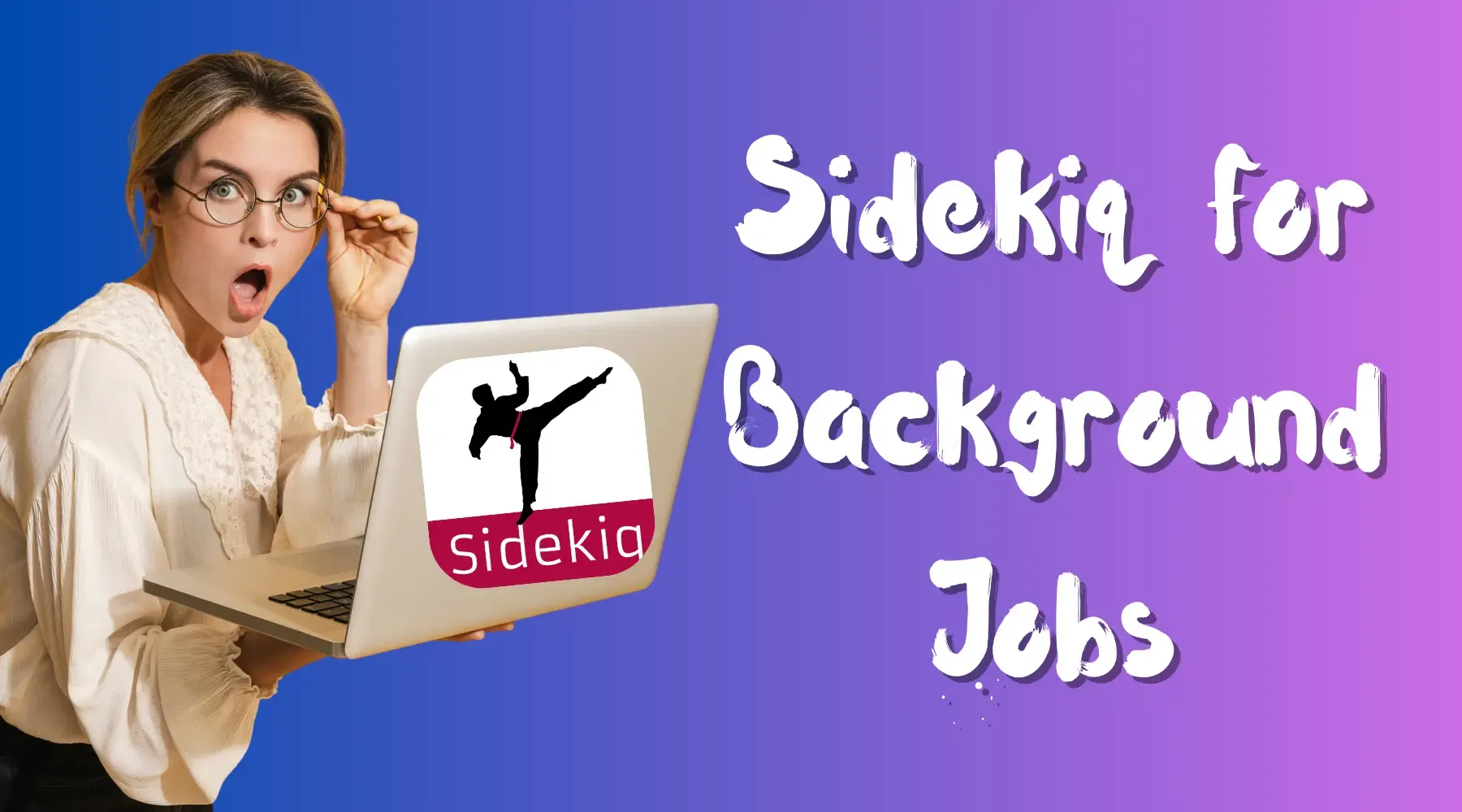
30-sec summary
As web applications grow, they often need to handle long-running tasks without slowing down user interactions. Sidekiq is a popular choice among Ruby on Rails developers for processing background jobs efficiently. This guide will cover what Sidekiq is, how to set it up in a Rails application, and provide practical examples to illustrate its usage.
What is Sidekiq?
Sidekiq is a background job processing library for Ruby. It allows developers to run tasks in the background, freeing up web requests to respond quickly to users. Unlike traditional job processors that may create separate processes, Sidekiq uses threads, which makes it lightweight and fast.
Key Features of Sidekiq
- 1.Concurrency: Sidekiq can process multiple jobs concurrently, making it efficient for handling large volumes of tasks.
- 2.Reliability: Jobs are stored in Redis, ensuring that they can be retried in case of failures.
- 3.Web Interface: Sidekiq offers a dashboard for monitoring jobs, making it easier to track progress and troubleshoot issues.
- 4.Scalability: As your application grows, you can add more worker processes to handle increased loads.
Setting Up Sidekiq in a Rails Application
Here's how to integrate Sidekiq into your Rails project step by step.
- 1.First, add the Sidekiq gem to your Rails application. Open your Gemfile and include the following line:
1gem 'sidekiq'
Run the following command to install the gem:
1bundle install
Next, you need to configure Sidekiq to connect to Redis. Create an initializer file for Sidekiq at config/initializers/sidekiq.rb and add the following code:
1require 'sidekiq'
2Sidekiq.configure_server do |config|
3 config.redis = { url: 'redis://localhost:6379/0' }
4end
5
6Sidekiq.configure_client do |config|
7 config.redis = { url: 'redis://localhost:6379/0' }
8end
9
Make sure you have Redis installed and running. You can check its status with:
1redis-cli ping
If it returns PONG, you’re good to go.
Create a Background Worker
Now, let’s create a worker that will define a job to be processed in the background. Generate a new worker using the following command:
1rails generate sidekiq:worker HardWorker
This will create a new file at app/workers/hard_worker.rb. Open that file and modify it like this:
1class HardWorker
2 include Sidekiq::Worker
3
4 def perform(name, count)
5 puts "Started work for #{name}..."
6 count.times do |i|
7 sleep(1) # Simulating a long-running task
8 puts "Processed task ##{i + 1} for #{name}"
9 end
10 puts "Completed work for #{name}!"
11 end
12end
Enqueue Jobs
With the worker created, you can now enqueue jobs to be processed. Here’s how you might do this from a controller:
1class TasksController < ApplicationController
2 def create
3 name = params[:name]
4 count = params[:count].to_i
5 HardWorker.perform_async(name, count)
6 render json: { status: 'Job is enqueued and will be processed in the background.' }
7 end
8end
Start Sidekiq
Now that everything is set up, you can start the Sidekiq process. Open a terminal and run:
1bundle exec sidekiq
Monitor Your Jobs
Sidekiq includes a web interface for monitoring jobs. To enable this, modify your routes.rb file:
1require 'sidekiq/web'
2Rails.application.routes.draw do
3 # Other routes...
4
5 mount Sidekiq::Web => '/sidekiq'
6end
Handling Job Failures and Retries
Sidekiq has a built-in retry mechanism for failed jobs. By default, if a job fails, Sidekiq will attempt to retry it several times before marking it as failed. You can customize this behavior using the sidekiq_options method in your worker class:
1class HardWorker
2 include Sidekiq::Worker
3
4 sidekiq_options retry: 3 # Set the number of retries
5
6 def perform(name, count)
7 raise "Simulated error!" if count < 0 # Simulate an error
8 puts "Started work for #{name}..."
9 count.times do |i|
10 sleep(1)
11 puts "Processed task ##{i + 1} for #{name}"
12 end
13 puts "Completed work for #{name}!"
14 end
15end
Custom Error Handling
If you want to add custom error handling, you can wrap your job logic in a begin-rescue block:
1class HardWorker
2 include Sidekiq::Worker
3
4 sidekiq_options retry: 3
5
6 def perform(name, count)
7 begin
8 raise "Simulated error!" if count < 0 # Simulate an error
9 puts "Started work for #{name}..."
10 count.times do |i|
11 sleep(1)
12 puts "Processed task ##{i + 1} for #{name}"
13 end
14 puts "Completed work for #{name}!"
15 rescue StandardError => e
16 logger.error "Failed to process job for #{name}: #{e.message}"
17 # Additional error handling (e.g., notifications) can go here
18 end
19 end
20end
Conclusion
Integrating Sidekiq into your Ruby on Rails application allows you to handle background jobs efficiently, keeping your application responsive even when performing resource-intensive tasks. With its ease of setup, robust features, and user-friendly monitoring interface, Sidekiq is a valuable tool for any Rails developer.
By following the steps in this guide, you should be able to implement Sidekiq in your application and manage background processing with confidence. As you continue to develop your Rails applications, consider how background job processing can enhance performance and improve user experience.